Building RAG Applications
Learn how to build a RAG application using Clarifai Python SDK
In the realm of text generation, Retrieval Augmented Generation (RAG) steps up the game for Large Language Models (LLMs) by fusing information retrieval capabilities with text generation skills, tackling key drawbacks of LLMs. When presented with a query, RAG fetches relevant information from an external knowledge base, which increases precision and contextual appropriateness through the integration of this retrieved data into the input. The Clarifai Python SDK allows you to create RAG-based applications with ease by reducing the number of steps in the process.
Click here to learn more about RAG.
Prerequisites
- Setting up the Clarifai Python SDK along with PAT. Refer to the installation and configuration with the PAT token here.
Guide to get your PAT
- Clone the Clarifai Examples repository to get the data files required for the building RAG.
!git clone https://github.com/Clarifai/examples.git
%cd /content/examples/
To run on a local system use: cd examples/
Before you proceed install llama_index
using pip install llama-index-core==0.10.24
Initialising RAG
The first part of creating a RAG-based application includes setting up the RAG object. Just by setting up the RAG object, Clarifai Python SDK will automatically create the app along with a prompter model and workflow containing the RAG prompter and the LLM Model.
- Python
# Import the RAG module from Clarifai for conversational AI tasks
from clarifai.rag import RAG
# Set the user ID for authentication
USER_ID = 'USER_ID'
# Define the URL of the Mistral-7B language model
LLM_URL = 'https://clarifai.com/mistralai/completion/models/mistral-7B-Instruct'
# Define a template string for generating prompts during inference
RAG_PROMPT_TEMPLATE = "<s>[INST] Context information is below:\n{data.hits}\nGiven the context information and not prior knowledge, answer the query.\nQuery: {data.text.raw}\nAnswer: [/INST]"
# Setup a RAG object with specified parameters such as user ID, model URL,
# minimum score threshold, and prompt template
rag_object = RAG.setup(user_id=USER_ID, llm_url=LLM_URL, min_score=0.5, max_results = 2, prompt_template=RAG_PROMPT_TEMPLATE)
Image Output
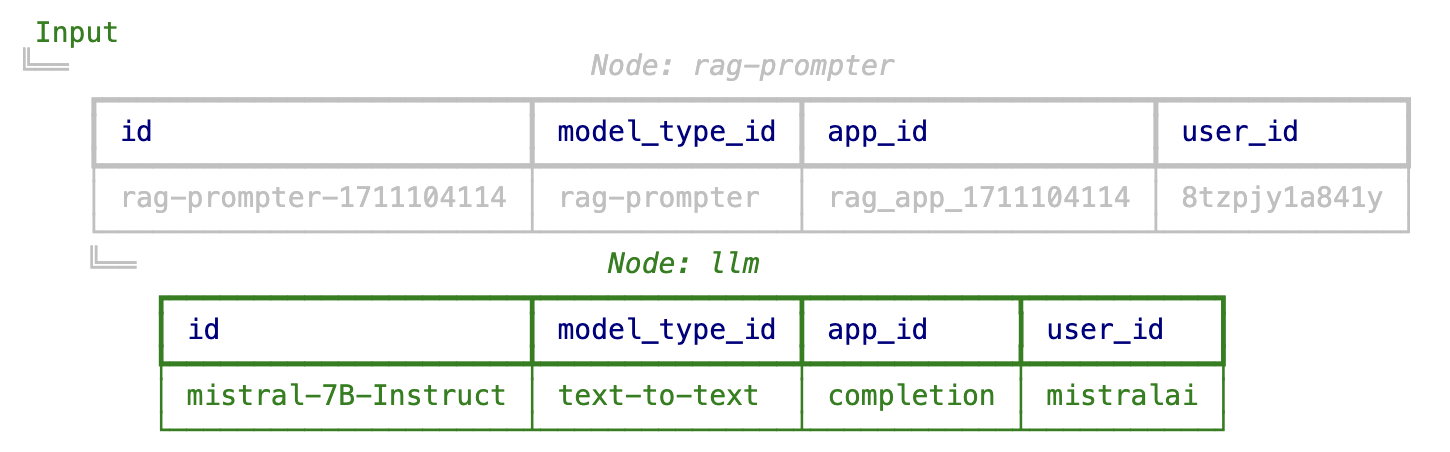
Here we are opting for Mistral-7B-Instruct as the LLM Model. You can choose different LLM Models for the RAG agent from Clarifai Community Models. The Clarifai Python SDK also allows you to set parameters like min_score,max_results and prompt_template for retrieving relevant data.
Dataset Upload
The next step involves uploading the dataset. In this example, we are using a Vehicle Repair Manual as data for the RAG. You can use the RAG object we created earlier for the data upload process. Now comes the perks of using Clarifai Python SDK. When you upload the data the Clarifai platform will automatically generate embeddings for the inputs and store them in the vector database which makes it ready for retrieval seconds after uploading data.
Supported formats for upload are Doc, PDF, Text, Folder Containing PDF, Doc and URL of PDF,Doc, Text files.
- Python
FILE_PATH="RAG/data/Crawfords_Auto_Repair_Guide.txt"
rag_object.upload(file_path=FILE_PATH,chunk_size= 1024) #parameters to split the document into chunks
Output
2024-03-20 10:34:02 INFO clarifai.client.input: input.py:674
Inputs Uploaded
code: SUCCESS
description: "Ok"
details: "All inputs successfully added"
req_id: "848c5233f9d67f1904da10c33a214ff9"
INFO:clarifai.client.input:
Inputs Uploaded
code: SUCCESS
description: "Ok"
details: "All inputs successfully added"
req_id: "848c5233f9d67f1904da10c33a214ff9"
Chat
In the final step, we are going to perform information retrieval using RAG based on the data we provided.
- Python
# Initiating a conversation with the RAG (Retrieval Augmented Generation) model object (`rag_object_gpt`).
# Sending a message containing the query "How to change brake fluid" to the model and awaiting a response.
result = rag_object.chat(messages=[{"role": "human", "content": "How to change brake fluid"}])
# Extracting the content of the response from the result.
answer = result[0]["content"]
# Printing out the response
print(answer)
Output
To change the brake fluid, you will need to follow these steps:
1. Locate the brake fluid reservoir in your vehicle. It is usually a clear plastic container with MAX and MIN markings on it.
2. Use a turkey baster or a brake fluid pump to remove the old brake fluid from the reservoir. Be careful not to spill any brake fluid on the car's paint as it can damage the finish.
3. Once the old fluid is removed, clean the reservoir with a lint-free cloth to ensure there is no contamination.
4. Refill the reservoir with new brake fluid that is recommended for your specific vehicle. Make sure to use the type of brake fluid specified in your owner's manual.
5. Slowly pour the new brake fluid into the reservoir up to the MAX marking. Avoid overfilling.
6. After filling the reservoir, you may need to bleed the brake system to remove any air bubbles. This process may vary depending on your vehicle, so it's best to consult your owner's manual or a professional mechanic for guidance.
7. Once the brake fluid is changed and the system is bled, check for any leaks or issues before driving the vehicle.
Remember, if you are not comfortable or experienced with changing brake fluid, it is recommended to have this task done by a professional mechanic. Brake fluid is a critical component of your vehicle's braking system, and proper maintenance is essential for your safety on the road.
Now let's ask questions that are related to the answer we received before so that we can be sure the RAG has understood the context properly.
- Python
result=rag_object.chat(messages=[{"role":"human", "content":"procedure after following the above steps"}])
answer=result[0]["content"]
print(answer)
Output
After following the steps to drain, flush, and pressure test the cooling system as described in the text, the next procedure would be to check for any leaks in the cooling system. This can be done by inspecting the entire cooling system, including the radiator, hoses, water pump, and heater core, for any signs of leaks. If there is less pressure on the gauge after the pressure test, there is probably a leak. Additionally, the engine should be started and the temperature gauge should be monitored to ensure that the cooling system is functioning properly. If the engine overheats or the temperature gauge reads high, further diagnosis and repair may be necessary.