Ranks
Learn how to perform search with ranks using Clarifai Python SDK
The rank feature in Clarifai allows users to specify criteria for prioritizing search results based on relevance or similarity to a reference. In the provided example, it conducts a vector search over inputs, comparing their features to those of a reference image specified by its URL. The search results are then ranked based on their similarity to the reference using the cosine metric.
Click here to know more about Rank search.
Rank with Text
By incorporating text-based ranking, users can enhance the relevance and specificity of search results, ensuring that items closely aligned with specified textual criteria appear higher in the ranked list.
- Python
from clarifai.client.user import User # Importing the User class from the Clarifai client library for user-related functionalities
from PIL import Image # Importing the Image module from the Python Imaging Library (PIL) for image processing
import requests # Importing the requests library to handle HTTP requests
from IPython.display import display # Importing the display function from IPython.display module for displaying images in IPython
USER_ID='' # Placeholder for user ID
APP_ID='' # Placeholder for application ID
PAT='' # Placeholder for personal access token (PAT)
# Initialize the User object with user ID and PAT
client = User(user_id=USER_ID, pat=PAT)
# Create a new application with specified ID and base workflow
app = client.create_app(app_id=APP_ID, base_workflow="Universal", pat=PAT)
# List of image URLs to be uploaded
urls = [
"https://images.pexels.com/photos/139257/pexels-photo-139257.jpeg",
"https://images.pexels.com/photos/1879386/pexels-photo-1879386.jpeg",
"https://images.pexels.com/photos/1071882/pexels-photo-1071882.jpeg"
]
input_obj = app.inputs() # Initialize Inputs object to manage input data
# Upload images from URLs to the application
for i, url in enumerate(urls):
input_obj.upload_from_url(input_id=f"input{i}", image_url=url)
# Initialize the search functionality for the application with top_k parameter set to 1
search = app.search(top_k=1)
# Perform a search query with a specified text rank
response = search.query(ranks=[{"text_raw": "Red pineapples on the beach."}])
# Extract the URL of the first hit from the search response
for r in response:
hit = r.hits[0].input.data.image.url
break
# Print the URL of the hit image
print(hit)
# Open the hit image from URL, resize it, and display it
hit_img = Image.open(requests.get(hit, stream=True).raw).resize((300,250))
display(hit_img)
Output
https://images.pexels.com/photos/139257/pexels-photo-139257.jpeg
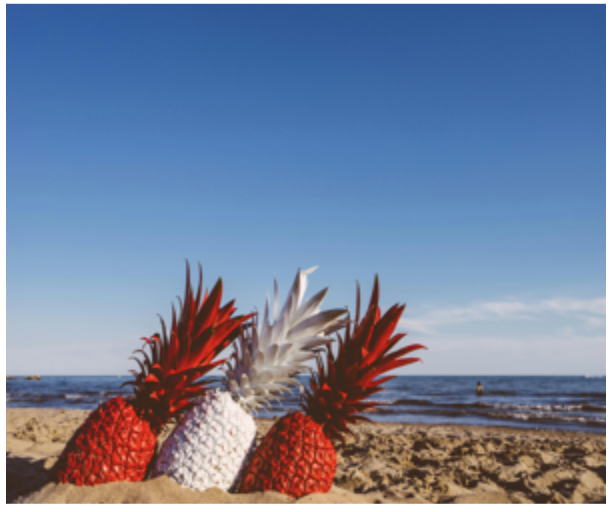
Rank with Image URL
Ranking with image URL in Clarifai allows users to prioritize search results based on the similarity or relevance of images specified by their URLs.
- Python
from clarifai.client.user import User
from PIL import Image
import requests
from IPython.display import display
# Replace these variables with your actual user ID, app ID, and PAT (Personal Access Token)
USER_ID = ''
APP_ID = ''
PAT = ''
# Initialize a User object with the provided user ID and PAT
client = User(user_id=USER_ID, pat=PAT)
# Create an application with the provided app ID, using the Universal workflow
# The PAT is also provided for authentication
app = client.create_app(app_id=APP_ID, base_workflow="Universal", pat=PAT)
# URLs of the images to be uploaded and searched
urls = [
"https://images.pexels.com/photos/139257/pexels-photo-139257.jpeg",
"https://images.pexels.com/photos/1879386/pexels-photo-1879386.jpeg",
"https://images.pexels.com/photos/1071882/pexels-photo-1071882.jpeg"
]
# Initialize an Inputs object to manage input data
input_obj = app.inputs()
# Initialize a Search object to perform searches
# Limit the number of returned results to 2 (top_k=2)
search = app.search(top_k=2)
# Upload each image from the provided URLs
for i, url in enumerate(urls):
input_obj.upload_from_url(input_id=f"input{i}", image_url=url)
# Perform a search with a specified rank (image URL)
res = search.query(ranks=[{'image_url': 'https://images.pexels.com/photos/139257/pexels-photo-139257.jpeg'}])
# Extract the URL of the first hit from the search results
for r in res:
hit = r.hits[0].input.data.image.url
break
# Print the URL of the hit image
print(hit)
# Open the hit image using PIL, resize it, and display it
hit_img = Image.open(requests.get(hit, stream=True).raw).resize((300, 250))
display(hit_img)
Output
https://samples.clarifai.com/XiJinping.jpg
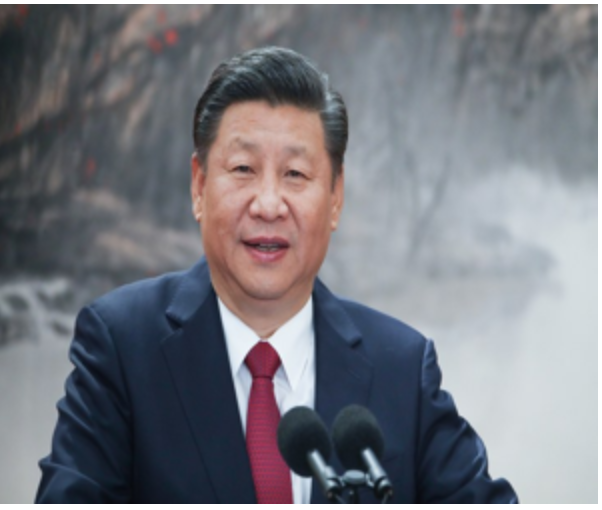